OOPs Concepts in Java
- There are 4 OOPs concepts in java:
- Inheritance
- Encapsulation
- Abstraction
- Polymorphism
- Inheritance: This is a special feature of Object Oriented Programming in Java.Inheritance called when child class acquires all properties and behaviors of parent class or superclass.
Class Super{
.....
.....
}
Class Base extends Super{
.....
.....
}
- Encapsulation: Encapsulation in Java is a mechanism for wrapping the data (variables) and code act on the data(methods) together as a single unit. For ex. capsule
- The Java Bean class is the example of fully encapsulated class.
An advantage of Encapsulation in java: By providing Getter and Setter methods we can make that class ad read-only or write-only.
- Example:
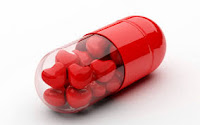
public Class Demo{
private Long id;
private String userName;
public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; }
}
- Abstraction: Abstraction means showing only functionality but how it works that will be hidden. For ex. In Bike when you give accelerate then it will go faster.But it will not show how it will go faster. So this is called abstraction.
- Abstract classes and Abstract methods :
- An abstract class may declare as an abstract keyword.
- An abstract method is a method that is declared without an implementation.
- It can be or cannot contain abstract methods.
- If a class is declared as an abstract class, it cannot be instantiated.
- Example:
class Employee
{
private int numOfEmployees = 0;
public void setNoOfEmployees (int count)
{
numOfEmployees = count;
}
public double getNoOfEmployees ()
{
return numOfEmployees;
}
}
public class EncapsulationExample
{
public static void main(String args[])
{
Employee obj = new Employee ();
obj.setNoOfEmployees(5613);
System.out.println("No Of Employees: "+(int)obj.getNoOfEmployees());
}
}
- Polymorphism: Polymorphism is a single action in different ways.For example, lets say we have a class
Animal
that has a methodbark()
, here we cannot give implementation to this method as we do not know which Animal class would extendAnimal
class. So, we make this method abstract like this:
public abstract class Animal{
...
public abstract void animalSound();
}
Now suppose we have two Animal classes
Dog
and Tiger
that extends Animal
class. We can provide the implementation detail there:
public class Dog extends Animal{
...
@Override
public void animalSound(){
System.out.println("Woof");
}
}
and
public class Tiger extends Animal{
...
@Override
public void animalSound(){
System.out.println("Roar");
}
}
- Types of Polymorphism:
- Static Binding or Compile time Polymorphism
- Dynamic Binding and Runtime Polymorphism
- Static Binding or Compile time Polymorphism:
In Compile time Polymorphism, a call is resolved by the compiler.It is also known as overloading and it is resolved at compile time.Overloading means A method having the same name but different parameters in the same class are known as overloading.
- Dynamic Binding or Run time polymorphism:
In Run time Polymorphism, a call is not resolved by the compiler.It is also known as overriding and it is resolved at runtime.Overriding means A method having the same name and same parameters in the different class using inheritance are known as overriding.
- Example:
Example of overloading
public class Car{
public void typeOfCar(){
System.out.println("Void method");
}
public void typeOfCar(String abc){
System.out.println("With parameter method");
}
}
Class Main{
public static void main(String[] args){
Car car = new Car();
car.typeOfCar();
}
}
Output:
Void method
Example of Overriding:
public class Car{
public void typeOfCar(){
System.out.println("Void method");
}
public void typeOfCar(String abc){
System.out.println("With parameter method");
}
}
public class Innova extends Car{
public void typeOfCar(){
System.out.println("Innova");
}
}
Class Main{
public static void main(String[] args){
Innova innova = new Innova();
car.typeOfCar();
}
}
Output:
Innova
Comments
Post a Comment